jQueryで簡単に実装!モーダルの作り方
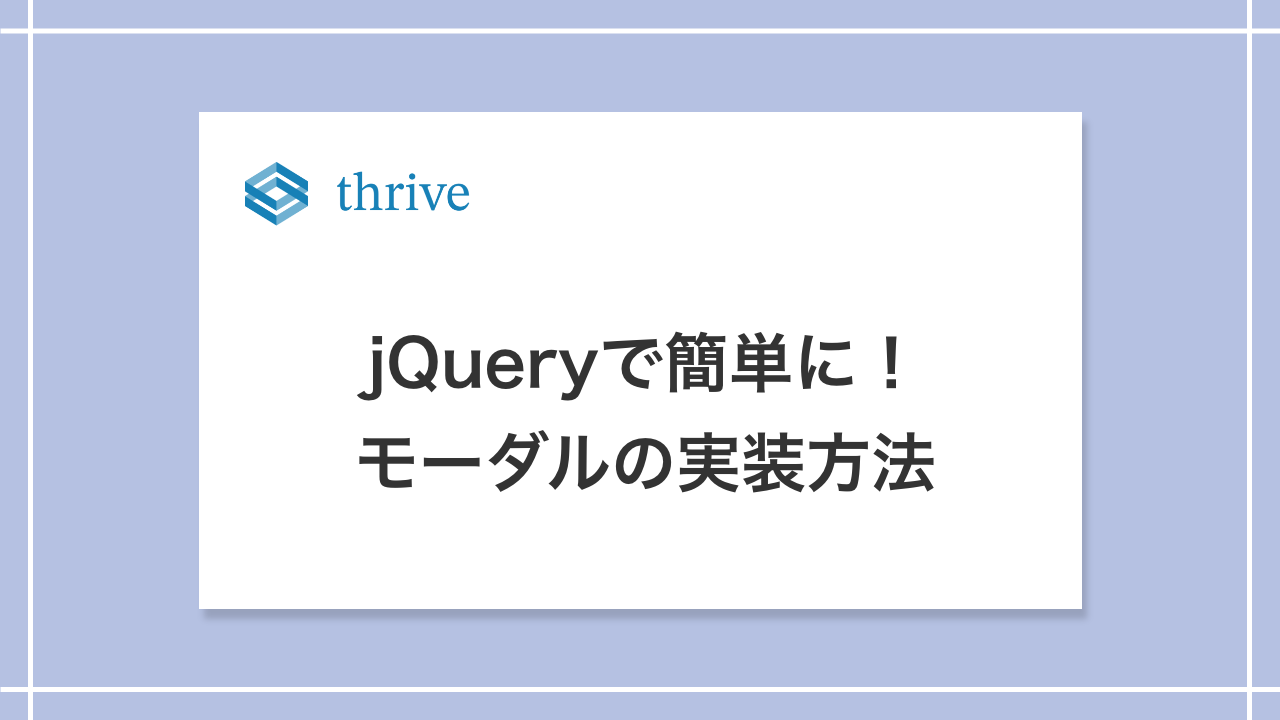
今回はシンプルで効果的なモーダルを作成する方法を紹介します。モーダルはユーザーエクスペリエンスを向上させる重要な要素であり、それを手軽に導入することができます。
デモ
See the Pen mofdal by hide (@hide225) on CodePen.
HTML構造の作成
あらかじめjQueryで操作する要素にidを指定します。
<!-- モーダルを開くボタン -->
<div class="modal-open" id="js-modal-open">モーダルを開く</div>
<!-- モーダル本体 -->
<div class="overlay" id="js-overlay">
<div class="modal">
<!-- 閉じるボタン -->
<div class="modal-close" id="js-modal-close">×</div>
<!-- モーダル内のコンテンツ -->
<div class="modal-content">
<p>コンテンツ内容。コンテンツ内容。コンテンツ内容。コンテンツ内容。コンテンツ内容。コンテンツ内容。コンテンツ内容。</p>
</div>
</div>
</div>
CSSスタイルの追加
activeクラスの付け外しで、表示・非表示の制御をするため、
付与している状態と、付与していない状態を作成します。
/*モーダルを開くボタン*/
.modal-open{
position: fixed;
display: flex;
align-items: center;
justify-content: center;
inset: 0;
width: 250px;
height: 50px;
color: #fff;
background-color: #333;
margin: auto;
cursor: pointer;
border-radius: 30px;
border: 1px solid #333;
transition: color ease .3s;
}
/*ホバーアニメーション*/
.modal-open:hover{
background-color: #fff;
color: #333;
}
/*モーダル背景の指定*/
.overlay{
position: fixed;
inset: 0;
width: 100vw;
height: 100vh;
text-align: center;
background: rgba(0,0,0,.7);
padding: 40px 20px;
overflow: auto;
opacity: 0;
visibility: hidden;
transition: .3s;
box-sizing: border-box;
}
/*モーダル本体の擬似要素の指定*/
.overlay:before{
content: "";
display: inline-block;
vertical-align: middle;
height: 100%;
}
/*モーダルにactiveクラスが付与したら*/
.overlay.active{
opacity: 1;
visibility: visible;
}
/*モーダル本体*/
.modal{
position: relative;
display: inline-block;
vertical-align: middle;
max-width: 450px;
width: 90%;
}
/*モーダルを閉じるボタン*/
.modal-close{
position: absolute;
top: 15px;
right: 15px;
width: 40px;
height: 40px;
texg-align: center;
line-height: 40px;
font-size: 25px;
color: #fff;
cursor: pointer;
background-color: #333;
border-radius: 50%;
}
/*コンテンツ内容*/
.modal-content{
background: #fff;
text-align: left;
padding: 50px 25px;
border-radius: 5px;
}
JQueryを使用しモーダルの表示・非表示を制御
最後にjQueryでactiveクラスの制御をします。
$(function(){
let open = $('#js-modal-open'),
close = $('#js-modal-close'),
container = $('#js-overlay');
//開くボタンをクリックしたらモーダルを表示する
open.click( function(){
container.addClass('active');
return false;
});
//閉じるボタンをクリックしたらモーダルを閉じる
close.click( function(){
container.removeClass('active');
});
//モーダルの外側をクリックしたらモーダルを閉じる
$(document).click( function(e) {
if(!$(e.target).closest('.modal').length) {
container.removeClass('active');
}
});
});
最後に
この手順に従えば、簡単にモーダルを実装することができます。ユーザーがボタンをクリックすると、モーダルが表示され、閉じるボタンをクリックすると非表示になります。ぜひ試してみてください!
関連記事
-
jQueryを使って簡単にカウントダウンタイマーを実装しよう
jQueryを利用すると、わずかなコードで素敵なカウントダウンタ ...
-
jQueryを使ったスムーススクロールの実装
この記事では、jQueryを使用してこのスムーススクロールを簡単 ...
-
jQueryを使用した簡単なローディング画面の実装方法
ウェブサイトやアプリケーションのユーザーエクスペリエンスを向上さ ...
-
Swiper.js基本的な使い方カスタマイズ方法
この記事では、Swiper.jsを使用してブログ記事のスライダー ...
-
初心者向け!jQueryを使った簡単なタブメニューの作り方
この記事では、jQueryを使用して簡単に実装できるタブメニュー ...
-
CSS + jQueryハンバーガメニュー実装方法
この記事では、CSSとjQueryを使用して、簡単かつ効果的にハ ...